Mastering the Fibonacci Series in C#: A Comprehensive Guide
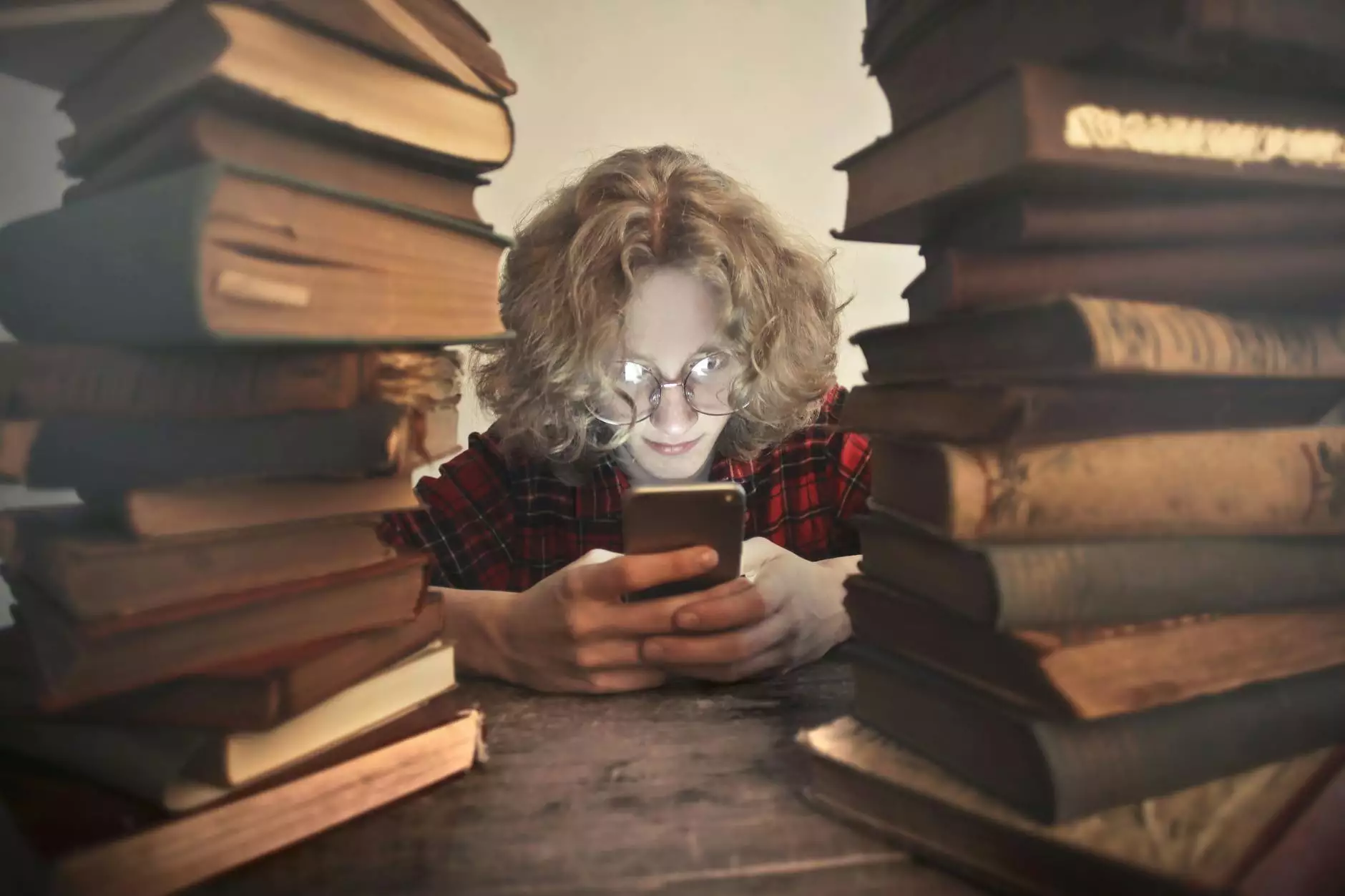
The Fibonacci series is not just a mere mathematical sequence; it represents a fundamental principle that permeates various aspects of nature, art, and even business. As an employment agency in South Africa, Kontak understands the importance of leveraging such mathematical concepts in programming, particularly in languages like C#. In this extensive guide, we will dive deep into how to accurately implement the Fibonacci series in C#, explore its significance, and discuss its applications in the business realm.
What is the Fibonacci Series?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. The sequence begins as follows:
- 0
- 1
- 1
- 2
- 3
- 5
- 8
- 13
This simple wholenumber pattern demonstrates growth and harmony, embodying principles that can be applied in various fields, including finance, biology, and computer science.
Implementing the Fibonacci Series in C#
When it comes to programming, implementing the Fibonacci series in C# can be achieved through several methods, including iterative, recursive, and using dynamic programming techniques. Below, we will explore each method in detail, empowering you to choose the best one for your needs.
1. Iterative Approach
The iterative method involves using a loop to calculate the Fibonacci numbers. This approach is efficient and easy to understand.
using System; class Fibonacci { static void Main() { int n = 10; // Fibonacci series length int first = 0, second = 1, next; Console.Write("Fibonacci Series: " + first + " " + second + " "); for (int i = 2; i < n; i++) { next = first + second; Console.Write(next + " "); first = second; second = next; } } }This code snippet creates a Fibonacci series of length ten. The use of variables makes the code readable and easy to modify.
2. Recursive Approach
The recursive approach, while less efficient than the iterative method, is elegant and closely mirrors the mathematical definition of the Fibonacci sequence.
using System; class Fibonacci { static void Main() { int n = 10; // Number of Fibonacci numbers to print for (int i = 0; i < n; i++) { Console.Write(FibonacciRec(i) + " "); } } static int FibonacciRec(int num) { if (numfibonacci series in c sharp 0789225888